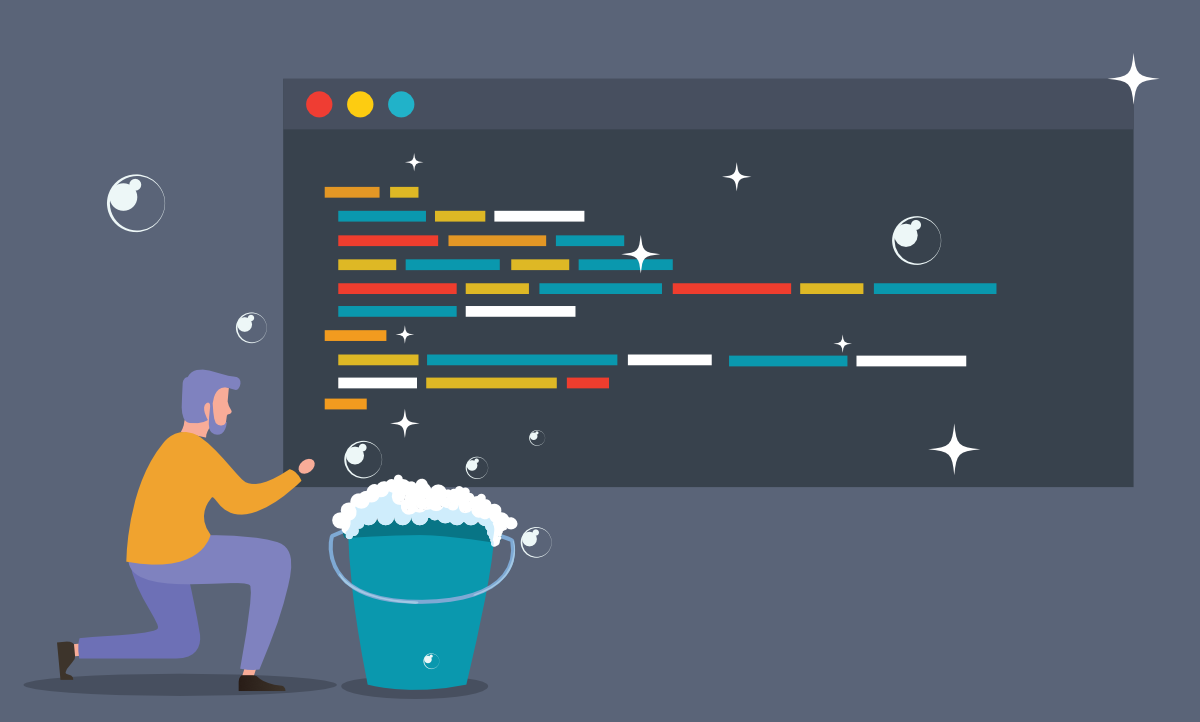
Powerful Tips to Help You Write Clean Code
Being a developer is more complex than many people realize.
It’s not simply about mastering a programming language and writing code to create software. There’s much more involved. One of the trickiest (and often most irritating) aspects for developers is clean code.
So, what exactly is clean code?
In short, it refers to writing code that’s so clear and well-structured that you or anyone else won’t struggle to understand it months down the line.
You can think of clean code as the programming version of excellent design, functional, aesthetically pleasing, and easy to work with.
I won’t go into detail about why clean code matters, you’re probably already aware of that. Instead, let’s jump right in and explore seven effective tips to help you write cleaner, more efficient code.
Table of Contents
- 1. Write Code Like You’re Explaining it to a 5-Year Old
- 2. Use AI Tools (or an AI Code Reviewer)
- 3. Get Rid of Unnecessary Comments
- 4. Follow the DRY Principle
- 5. Fix Your Code Formatting & Follow a Consistent Style
- 6. Don’t Let Your Functions Do Too Much
- 7. Organize Your Files and Folders Properly
- Wrapping Up
1. Write Code Like You’re Explaining it to a 5-Year-Old
Let’s be honest, if you’re writing overly complicated code that others—whether on your team or elsewhere—can’t easily understand, it’s not fulfilling its purpose.
Your objective should be to write code so simple that even someone who’s seeing the file for the first time can quickly grasp what’s happening.
For example, if your variable names are something like this:
let a = b + c;
This isn’t helpful. No one will know what a
, b
, or c
represent—even you, a few weeks down the road.
Variables should clearly describe what they hold, essentially acting like self-explanatory comments. Here’s a better example:
let totalAmount = productPrice + shippingFee;
This simple approach should be applied everywhere—functions, comments, and more.
Here’s an example of code that’s difficult to understand:
function compute(cartItems) {
let t = 0;
for (let i = 0; i < cartItems.length; i++) {
t += cartItems[i].cost;
}
return t;
}
This only gives a vague sense of what the function does based on the logic, but not much else.
Instead, rewrite it like this:
function calculateTotal(cartItems) {
let totalAmount = 0;
for (let i = 0; i < cartItems.length; i++) {
totalAmount += cartItems[i].price;
}
return totalAmount;
}
Now, just by glancing at the code, it’s clear what’s happening!
2. Use AI Tools (or an AI Code Reviewer)
AI is advancing rapidly and is becoming a part of nearly every industry.
As a developer, you can take advantage of AI to help write clean and understandable code—thanks to AI tools like ChatGPT, Claude, and GitHub Copilot.
For example, you can copy and paste your code into a large language model (LLM) and ask it to review your code.
Here’s an example of a request I made to ChatGPT:
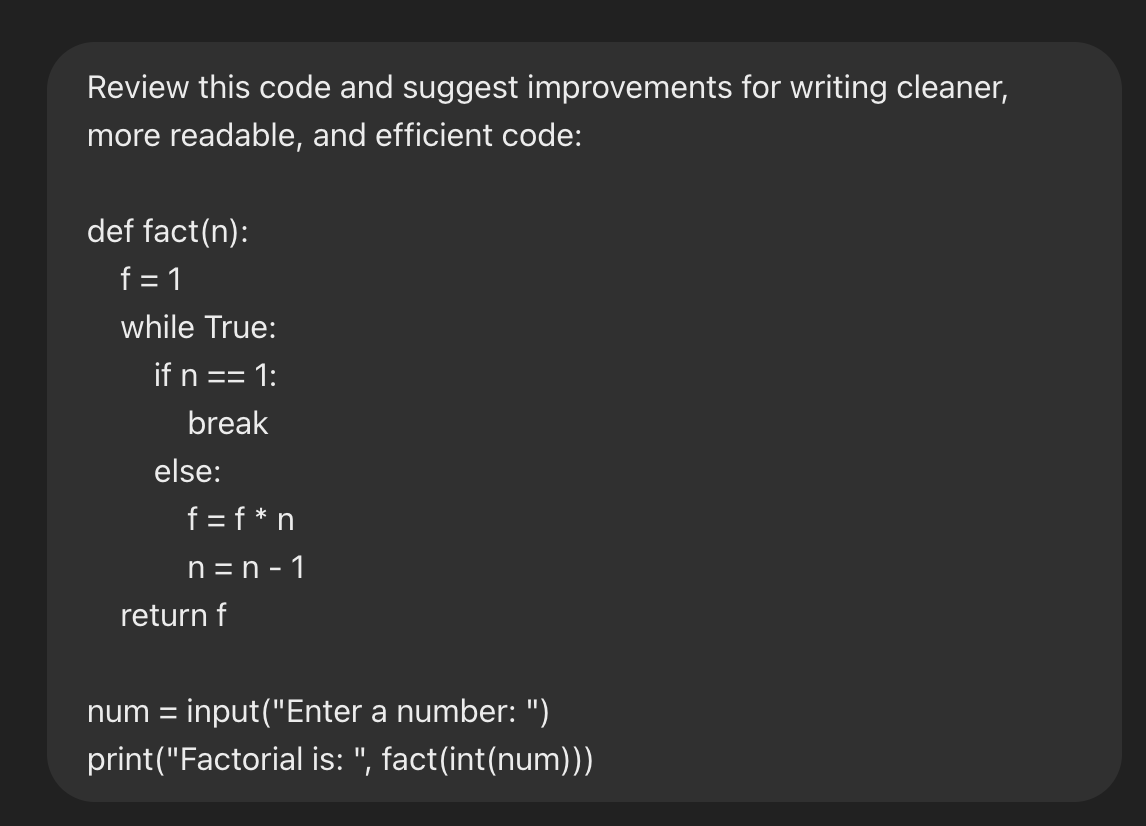
And here’s what ChatGPT suggested:
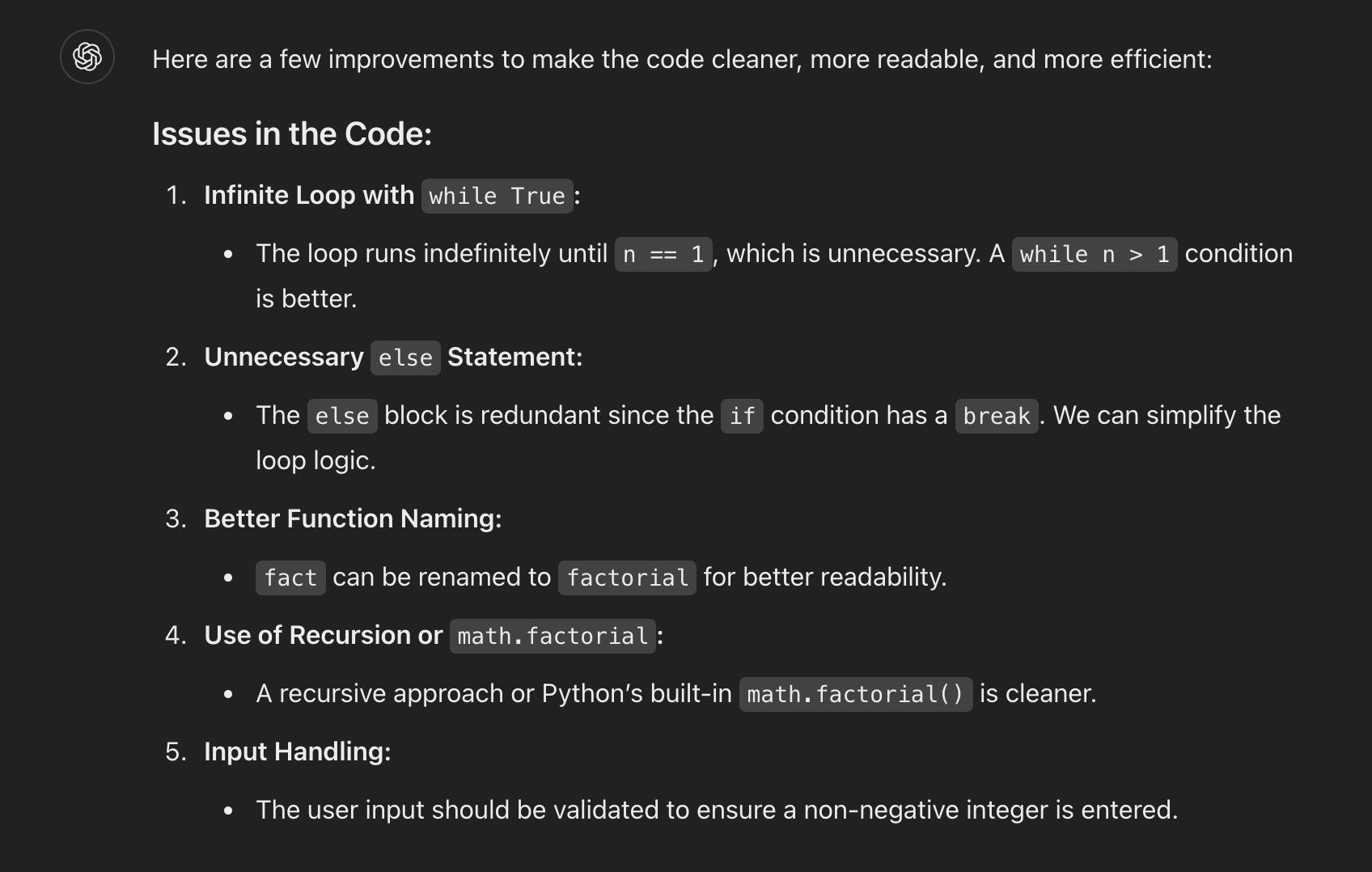
It even provided an improved version of my code, which looks like this:
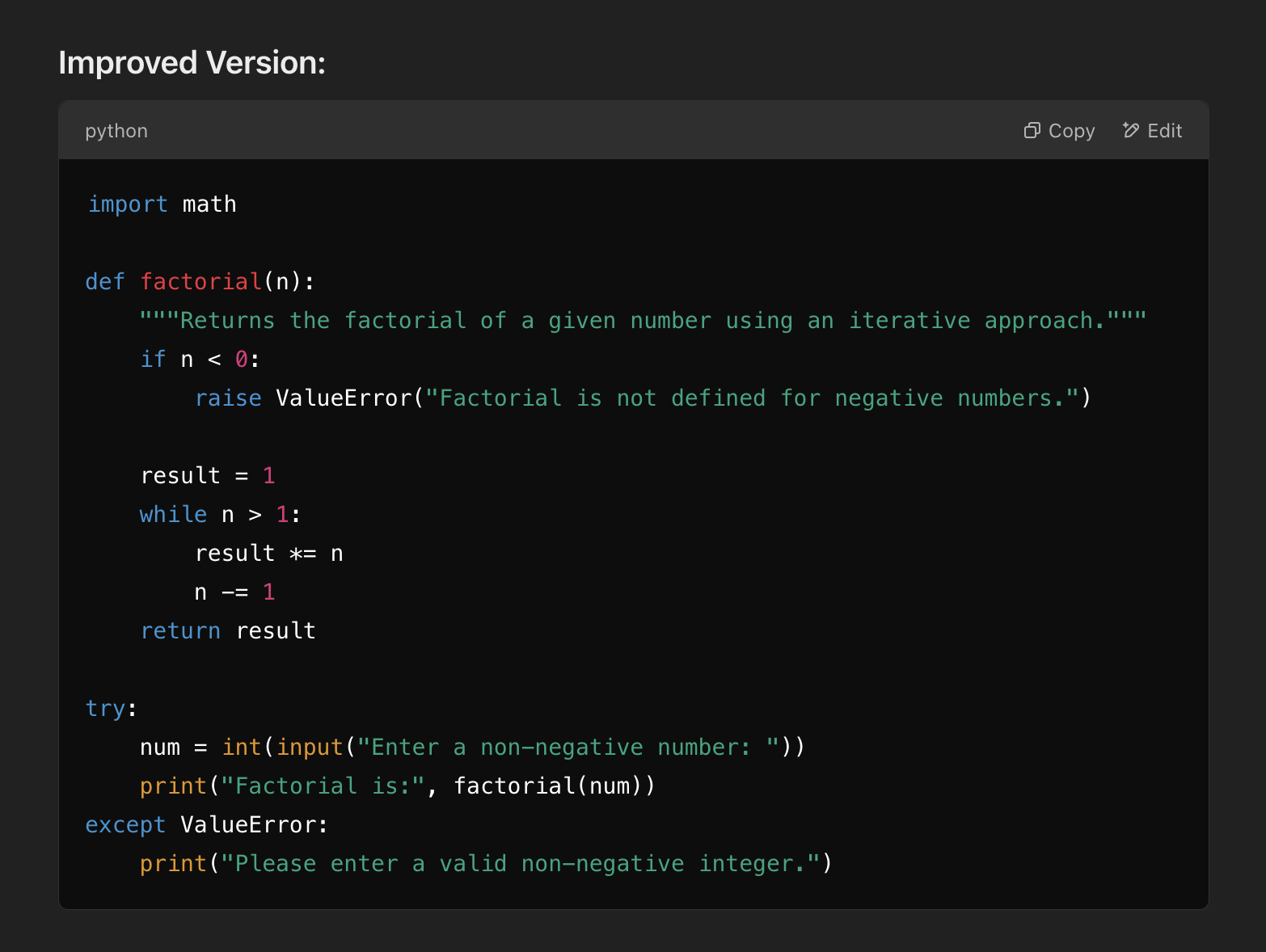
Additionally, you can use AI-driven code reviewers like CodeRabbit AI. It integrates with your pull requests and offers automated code reviews, explanations, and much more.
In simple terms, when you install CodeRabbit AI, add it to your pull request, and create a pull request, it provides you with summaries, reviews, walkthroughs, and more.
If you want to learn more, here’s a quickstart guide to help you get started with CodeRabbit AI.
Keep in mind that while AI tools are great for improving your code, they shouldn’t replace your own judgment.
Whenever AI suggests changes, always ask yourself: Does this make sense?
AI isn’t perfect—it can generate faulty code and might miss important details that only a human can fully comprehend.
Instead of blindly accepting AI’s suggestions, take a moment to understand why they were made.
The goal is to use AI to speed up your workflow, catch mistakes, and assist with other tasks, while you maintain full control over your code.
3. Get Rid of Unnecessary Comments
Writing useful code comments can be helpful in explaining why your code does what it does.
However, I often see developers writing excessive comments, even in situations where they aren’t necessary.
My belief is that good code should, whenever possible, document itself.
Here’s an example of a comment that doesn’t add much value:
// Adding 10 to the result
totalAmount = totalAmount + 10;
In this case, the comment doesn’t really add anything to the understanding of the code.
It’s better to write comments only when they’re necessary to help a reviewer understand the reasoning behind your approach or when there’s something unclear that needs an explanation.
Here’s a better example:
// Adding 10 because the client requires a 10% buffer for calculations
totalAmount = totalAmount + 10;
Now the comment clearly explains why the developer added 10 to the total amount, providing useful context for anyone reviewing the code.
4. Follow the DRY Principle
I’ve noticed many programmers repeating the same logic or adding identical functionality across different files.
There’s no need to duplicate the same code over and over, as it only complicates things. Keep your code DRY (Don’t Repeat Yourself).
Instead, abstract that logic into a single, reusable function.
For example, instead of repeating the same logic in different places like this:
if (person.age > 18 && person.age < 65) {
// Perform some action
}
if (person.age > 18 && person.age < 65) {
// Perform another action
}
You can create a reusable function and use that logic wherever needed:
function isAdultWorkingAge(age) {
return age > 18 && age < 65;
}
if (isAdultWorkingAge(person.age)) {
// Perform some action
}
In short: write it once, and use it everywhere.
5. Fix Your Code Formatting & Follow a Consistent Style
This is another simple but often overlooked tip.
First, make sure your code is properly indented and formatted.
You can easily set up a VS Code extension or a linter like Prettier, ESLint, or Flake8, depending on your programming language. After configuring it, you’ll be good to go.
These linters not only help you write better code by catching mistakes and enforcing coding standards, but they also improve consistency, catch errors early, and make your code easier to read. This ultimately saves time on bug fixes and code reviews.
However, improving your formatting is just one piece of the puzzle—it’s about more than just clean code.
In addition to formatting, you should stick to a consistent style guide for things like function names, variable names, and other coding conventions.
For example, here’s some code with inconsistent styling:
let total_amount;
let UserInformation;
function fetchdata() {}
Why is this inconsistent?
It mixes different naming conventions—total_amount
uses snake_case, UserInformation
uses PascalCase, and fetchdata()
uses lowercase instead of camelCase.
This makes your code harder to follow and more confusing. Instead, adopt a consistent style like this:
let userInformation;
let totalAmount;
function fetchData() {}
For JavaScript, camelCase is the standard for variables and functions, so fetchData()
, userInformation
, and totalAmount
are more consistent.
Whether you’re working solo or in a team, adhering to one style makes your code cleaner and easier for anyone to review.
6. Don’t Let Your Functions Do Too Much
As a programmer, I understand that sometimes you need to write complex logic in a function.
However, more often than not, developers cram too much logic into a single function, making it handle multiple responsibilities. These functions become too complex and hard to read or understand.
It’s much better to create several smaller functions, each with a single responsibility.
Here’s an example of a function that tries to do too much:
function calculateCart(cartItems) {
let total = 0;
for (let i = 0; i < cartItems.length; i++) {
total += cartItems[i].price * cartItems[i].quantity;
}
return total > 100 ? total * 0.9 : total;
}
This function is overloaded—it both calculates the total price based on quantity and applies a discount if the total exceeds a certain amount. This makes it harder to reuse for specific purposes.
A better approach is to break the logic into three separate functions:
- One function to calculate the total price based on quantity.
- One function to apply the discount.
- One function to get the total price and apply the discount if needed.
This results in cleaner, more manageable code. Here’s how it could be refactored:
function calculateCart(cartItems) {
const total = getCartTotal(cartItems);
return applyDiscount(total);
}
function getCartTotal(cartItems) {
return cartItems.reduce((sum, item) => sum + item.price * item.quantity, 0);
}
function applyDiscount(total) {
return total > 100 ? total * 0.9 : total;
}
As you can see, instead of cramming everything into one long function, the logic is broken into smaller, clearer functions.
This makes the code more understandable and easier to maintain, both for you and others.
7. Organize Your Files and Folders Properly
Now we’re getting to one of the most important aspects.
When working on a large project, dumping all your code into a single folder with random files is a recipe for disaster. It becomes a nightmare for anyone reviewing the code or trying to find a specific file.
Imagine this—going through 50 files just to fix a single bug. No one wants that.
Instead, organize your project into multiple folders based on features or pages.
And don’t stop there—break down large files into smaller, more manageable modules based on their functionality. This way, everything is neat, easy to navigate, and instantly makes sense.
Here’s an example of a poorly structured project:
plaintextCopierproject-folder/
│
├── index.html
├── app.js
├── helpers.js
├── data.js
├── user.js
├── product.js
It’s messy, right? Now, here’s a more organized approach:
plaintextCopierproject-folder/
│
├── pages/
│ ├── home/
│ │ ├── HomePage.js
│ │ ├── HomePage.css
│ │ └── HomePage.test.js
│ ├── user/
│ │ ├── UserPage.js
│ │ ├── UserPage.css
│ │ └── UserPage.test.js
│ └── product/
│ ├── ProductPage.js
│ ├── ProductPage.css
│ └── ProductPage.test.js
├── components/
│ ├── Header.js
│ ├── Footer.js
│ └── Button.js
├── utils/
│ └── api.js
└── index.js
Now, see how much simpler this is?
You have distinct folders for pages, components, and utilities. Inside each folder, files are clearly organized by their function and given clear, descriptive names.
For example, if you’re looking for something related to the product page, you’ll know exactly where to find it. This organization helps maintain clarity and makes your project easier to manage.
Wrapping Up
In this article, we covered the fundamentals you need to start writing clean, efficient, and maintainable code.
We discussed essential practices such as writing clean code, utilizing AI tools, applying proper code formatting, structuring functions effectively, and following other best practices.
By consistently applying these tips, you’ll greatly enhance your coding skills and produce cleaner, more readable code.
I hope you found this helpful!